Assignment 8: Trust the Process
Due Friday, November 1, at midnight
The goals for this assignment are:
-
Gain experience working with
fork()
. -
Use OS system calls in your C program.
-
Implement your own simple shell program.
Update your repository
Do a fetch upstream to obtain the basecode for this assignment.
Using the command line
-
Open terminal and change your current directory to your assignment repository.
-
Run the command
git fetch upstream
-
Run the command
git merge upstream/master
Your repository should now contain a new folder named A08
.
The fetch
and merge
commands update your repository with any changes from the original.
1. Warm-up
In the file, warmup.c
, implement a program that spawns processes according to the following diagram.
![]](img/processes.png)
$ make warmup
gcc -g -Wall -Wvla -Werror warmup.c -o warmup
$ ./warmup
17300] A0
17300] B0
17301] B1
17301] Bye
17300] C0
17300] Bye
17302] C1
17302] Bye
Requirements:
-
Your program should print the messages as shown above.
-
Your program should use fork() to spawn processes.
-
Call
getpid()
to show the process ids for each print message.
2. Personal Shell
In the file, shell.c
, implement a program that implements a simple shell.
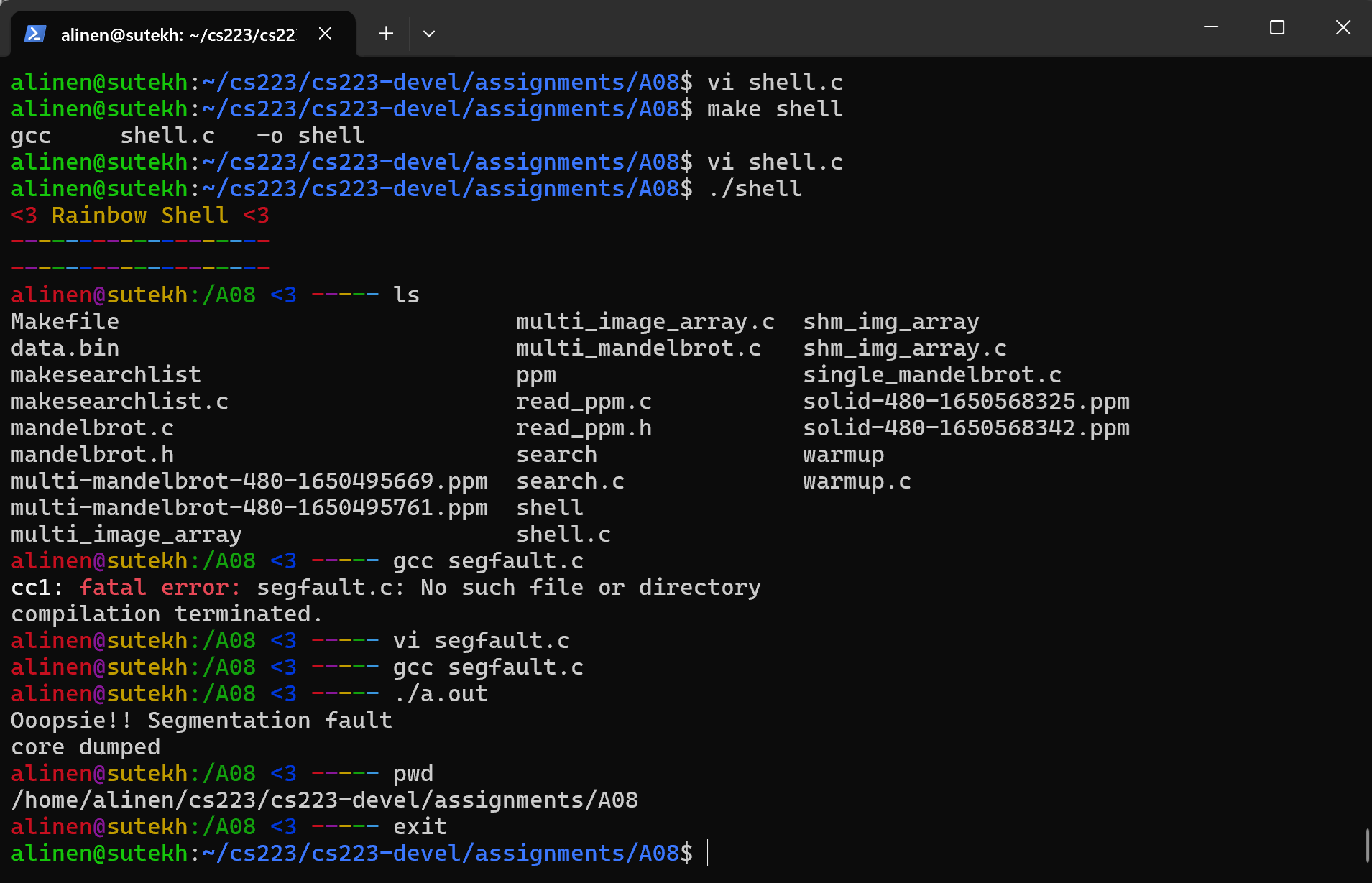
Requirements:
-
Your program should print a prompt. At minimum, your prompt should show the current working directory and look distinct from the lab machine prompts. In other words, it should be easy to tell what shell if running from the terminal.
-
Use the
readline()
function to get user input. This function supports backspace, tab completion, and more. Documentation for readline can be found here. -
Use the
add_history()
function to save user history. This will allow arrow keys to work. Documentation for readline can be found here. -
Your program should quit if the users types
exit
. -
When the user gives a command, split it into a command and arguments and then fork a command to execute it.
-
Your terminal should wait for the command to finish. If the command terminates with an error, report the error.
readline is a package that is installed on goldengate and in our labs. However, you may need to install it on your own machine. On Unix systems, this can be done by running sudo apt install libreadline-dev
|
You can print with colors to the console using ANSI escape sequences (see below).
For example, printf(ANSI_COLOR_GREEN "Green Text" ANSI_COLOR_RESET); will print green text. Here is a good guide!
|
// Helpful macros for working with color #define ANSI_COLOR_RED "\x1b[31m" #define ANSI_COLOR_GREEN "\x1b[32m" #define ANSI_COLOR_YELLOW "\x1b[33m" #define ANSI_COLOR_BLUE "\x1b[34m" #define ANSI_COLOR_MAGENTA "\x1b[35m" #define ANSI_COLOR_CYAN "\x1b[36m" #define ANSI_COLOR_RESET "\x1b[0m"
Most prompts print helpful information. Above, we use the
system commands gethostname , getcwd , and getpwuid(geteuid()) to get the
machine name, the current working directory, and current user respectively.
|
3. Submit your work
Push your code to github to submit your work
$ git status
$ git add .
$ git status
$ git commit -m "assignment complete"
$ git status
$ git push
$ git status
4. Grading Rubric
Assignment rubrics
Grades are out of 4 points.
-
(1 points) Warmup
-
(0.1 points) style and header comment
-
(0.4 points) prints correct messages, uses
fork()
andgetpid()
-
(0.5 points) no memory errors
-
-
(3 points) Simple shell
-
(0.1 points) style and header comment
-
(0.7 points) Displays unique prompt with current working directory
-
(0.3 points) Uses
readline()
andadd_history()
-
(0.1 points) Quits when the user types
exit
. -
(0.1 points) Forks a process to run the user’s command.
-
(0.1 points) Reports the return status of the command when the child process completes.
-
(1.5 points) no memory errors.
-
When using readline() , valgrind will report that some of your memory is
still reachable. This memory is not leaked. It hasn’t been cleaned up yet from
`readline()’s internal memory. For example, output such as the following is ok
|
==3504== HEAP SUMMARY: ==3504== in use at exit: 204,143 bytes in 221 blocks ==3504== total heap usage: 453 allocs, 232 frees, 244,790 bytes allocated ==3504== ==3504== LEAK SUMMARY: ==3504== definitely lost: 0 bytes in 0 blocks ==3504== indirectly lost: 0 bytes in 0 blocks ==3504== possibly lost: 0 bytes in 0 blocks ==3504== still reachable: 204,143 bytes in 221 blocks ==3504== suppressed: 0 bytes in 0 blocks
Code rubrics
For full credit, your C programs must be feature-complete, robust (e.g. run without memory errors or crashing) and have good style.
-
Some credit lost for missing features or bugs, depending on severity of error
-
-5% for style errors. See the class coding style here.
-
-50% for memory errors
-
-100% for failure to checkin work to Github
-
-100% for failure to compile on linux using make